The daily email madness can sometimes be exhausting. So it’s good to be able to simplify and automate your work with a few VBA macros. One use case could be that you have marked one or more e-mails and want to mark them as read in one go using VBA code.
This use case will rarely occur “standalone“. In most cases, it will be used in conjunction with other macros, e.g. if you want to mark e-mails as read and then automatically move them to another folder. Or you want to reply to an e-mail automatically using a VBA macro and then mark it as read.
Macro mark email as read
In the following image and inserted code, we can already see how small and inconspicuous the code is.
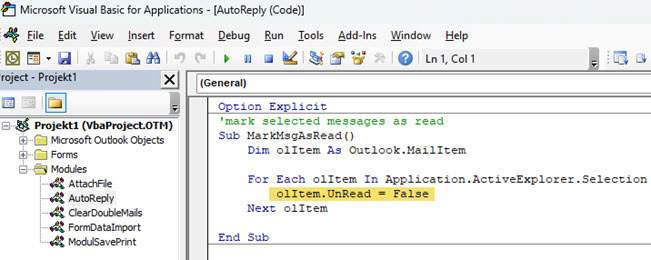
Option Explicit.
'mark selected messages as read.
Sub MarkMsgAsRead()
Dim olItem As Outlook.MailItem
For Each olItem In Application.ActiveExplorer.Selection
olItem.UnRead = False
Next olItem
End Sub
So there is a method MarkMsgAsRead which can then be executed from Outlook as a macro. Within the method, an olItem (short for outlookItem) is defined as an MailItem. In a For-Each loop, all emails selected in the inbox are then run through. In order to mark the MailItem as read, the property “UnRead” must be assigned the value “False”.
Macro: mark email as unread
Of course, the whole thing also works in reverse, so that you can mark e-mails that have already been marked as read as unread. To do this, simply set the “UnRead” property to “True”.
Option Explicit
'mark selected messages as unread.
Sub MarkMsgAsUnRead()
Dim olItem As Outlook.MailItem
For Each olItem In Application.ActiveExplorer.Selection
olItem.UnRead = True
Next olItem
End Sub
Note
A final note: If you get error messages the first time you run the code, just check that you have selected the correct references/libraries. These can be found in the menu under Tools ➔ References.
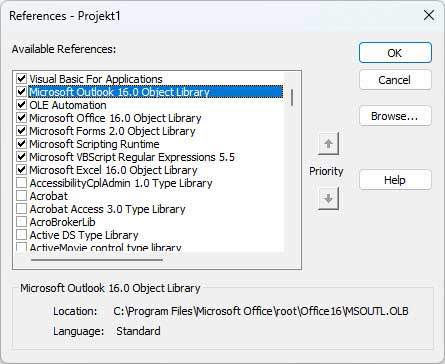